I Wrote a VS Code Extension to Sort Your Flutter Project’s Imports
Easily sort your dart imports
TL;DR
The Dart Import Sorter extension does one main thing: It converts your imports from a mess to something neat and consistent.
Intro
For the most part, IDEs take care of organizing your imports for you. I’m not an avid IntelliJ user but I often appreciated the way it just keeps imports out of sight and out of mind.
I wanted the same functionality in VS Code but I couldn’t find any extension in the market to achieve the behavior that I wanted. So, like any overly-ambitious programmer, I decided to write my own!
Description
The Dart Import Sorter extension does one main thing: It converts your imports from a mess to something neat and consistent.
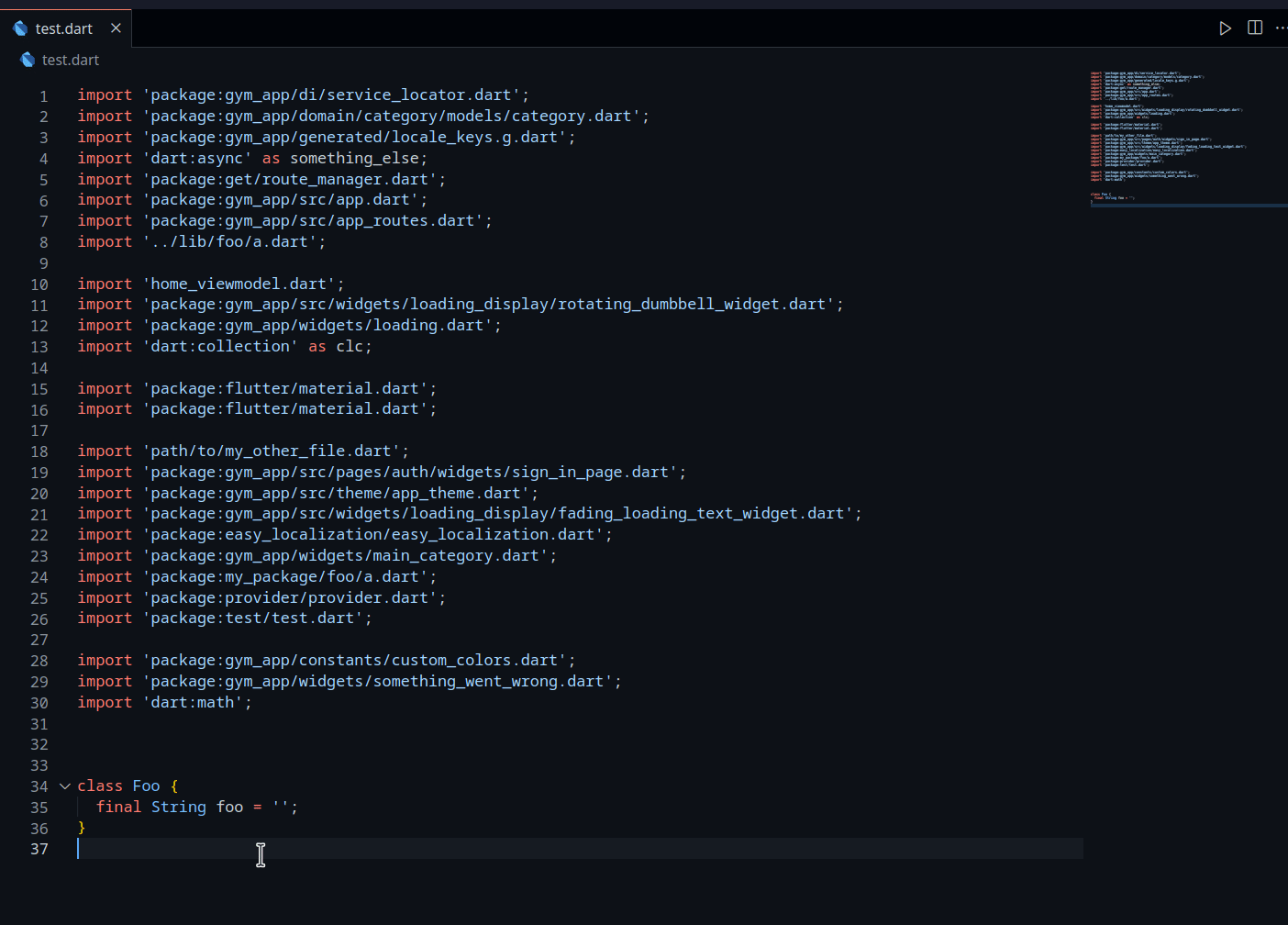
Setting up your own config
You can either go with the default config or you can customize it to suit your needs. The extension can be configured using regular expressions, so sky’s the limit.
Here’s an example configuration:
According to the example above, the extension will look for imports matching the Flutter rule, then Dart, then Everything Else. These labels aren’t actually used in the sorting process. They’re just here to help you keep track of what each rule does.
Here’s a demo of these rules. The following is a bunch of messy imports:
The above code becomes like the following after being sorted:
You’ll notice new lines between each group and that imports within groups are sorted alphabetically.
You can turn off having new lines between groups by setting the following rule:
"dartimportsorter.leaveEmptyLinesBetweenGroups": false
How to use
You can run the extension using the shortcut Ctrl + Alt + O
, or you can use the command palette Ctrl + Shift + P -> Dart: Sort Imports
.
Finally, you can set the extension to sort your imports when you save your file. To do that, set the following rule:
"dartimportsorter.sortOnSave": true
Why sort your imports?
When I first started writing this extension, it was really because I just liked seeing my imports grouped and organized. But then, I found a couple of (sort of) compelling reasons:
- Your imports are more compact at the beginning of your file. You know which part to skip immediately to get to the actual code. You can also collapse the whole block to make it easier.
- You can figure out and fix incorrect imports quicker if such a need arises.
About the extension
Note: This part doesn’t really talk about the functionality of the extension, but rather the development process.
I’ve used the process of developing this extension as a bit of a playground to try a few programming/architecture techniques that I’ve been reading about. Mainly Clean Architecture (by uncle bob). I haven’t really applied it here but it’s inspired me to apply the SOLID principles (or at least give it a good try).
I managed to implement inversion of control by injecting dependencies using TSyringe. This allowed me to decouple things from each other and properly do unit tests without involving the vs code extension API.
I’m not the most experienced person when it comes to writing tests but I have to admit, having tests seriously increased my confidence in the changes that I’ve implemented in my code, whether it’s adding features or refactoring. All I need to do to make sure all’s well is just to write a little command in bash and if it lights up green then I’m good to go.
If you’re interested in contributing or you’d like to ask for a feature, here’s the link to the repo
Also, here’s a link to the extension in VS Code marketplace
Happy Coding!