React Context API + Hooks. Part 2 — UI Language Switch
Changing UI language with Hooks and Context
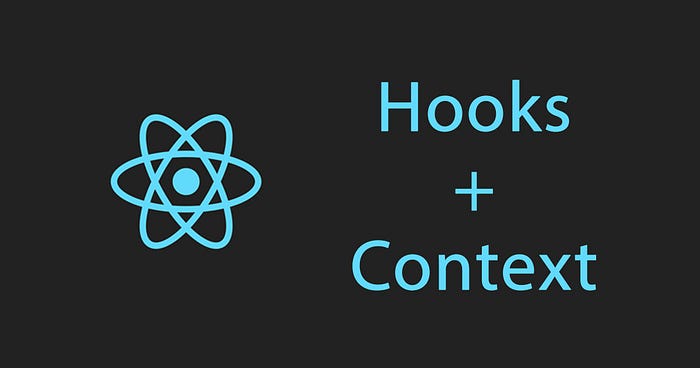
In this post, we’ll continue to explore the usage of the React Context API and how you can add multiple UI languages to your React app.
If you’ve read my previous post, you’re familiar with how Context works and why we need it. If not, read: React Context API. Part 1 — Dark Theme.
1. Create a Simple App
Start by creating a simple app. This app will display information about movies.
We’ll mock an API fetch request, to keep the app simple and focus on the feature implementation. This time, we don’t care about CSS.
2. Create a Context File
As in the previous post, we initialize a new Context by calling a React.createContext
function which takes an object as an argument.
The object stores values which will be updated by the Provider later. It could be empty, but it’s good practice to have the keys with empty values for testing and autocompletion purposes.
const LangContext = React.createContext({
lang: '', // for currently used language
currentLangData: '', // lang data (names, titles)
switchLang: () => {},
)};
In LangProvider
, we need to get the user’s browser’s current language and save it in the Provider’s state.
In the event that the browser’s language is different from what the user prefers, we will save the last chosen language to the local storage. We’ll prioritize it over the default browser’s language using the ||
operator so that, on the next page load, the app will render the right one.
We use the useLayoutEffect
Hook because the app needs to know which language to display before the initial render. The second argument of useLayoutEffect
accepts an array of dependencies for the effect.
Later, on a button click, we’ll call switchLang()
which updates the state (lang). This gives useLayoutEffect
a signal to re-render the app, passing new currentLang
data to components which consume that data and render the title in the current language.
To retrieve the data of the currently chosen language, we dynamically set it in the value object of the Provider. We also need an object to store the titles in different languages.
This is what it looks like all together:
Let’s refactor Card.js
, by subscribing to the context changes and replacing the hardcoded titles for dynamic values.
In LangSwitch
, we pass the switchLang
function from the context to the onClick
button handler.
We can also conditionally render the button className
, so we know which language is currently active.
When a user changes the UI language, we want to fetch new data from the API based on the chosen language. To do that, we’re passing the current lang
from the Context’s state to the fetchMock
as an attribute in App.js
.
In the real API request, we would pass it as a query parameter or make a request to a different endpoint, depending on the API architecture.
Also, we pass the lang
as a second attribute to useEffect()
, so it knows when to re-fetch. Then, we change the h1
to a dynamic value.
And that’s it! I hope you enjoyed it.