How To Use Jenkins for Automated Builds of Docker Images
A guide to creating a Jenkins job to build and publish images on Docker Hub
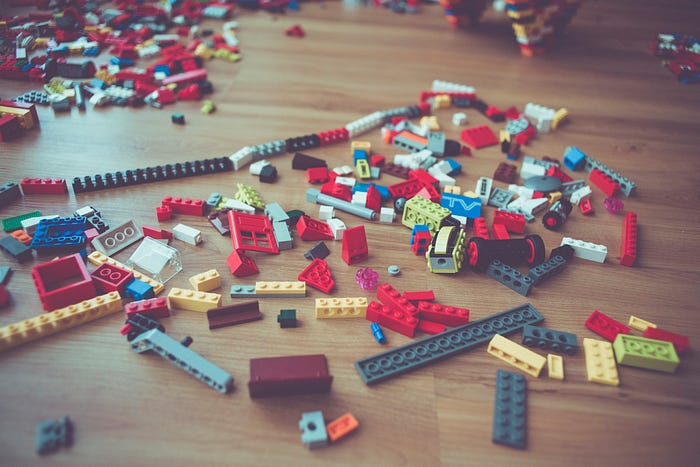
Build jobs are at the heart of the Jenkins build process. You can think of a Jenkins job as a specific task or step in your build process. A build can involve compiling your source code, running tests, packaging it, and publishing it.
In this article, we will create a Jenkins job that:
- Infers remote branches of a given project
- Compiles the code
- Packages the code using Docker
- Publishes the image to the Docker Hub
By the end of this tutorial, you should have something like the following:

Create a Job in Jenkins
From the main Jenkins page, create a new item by clicking on New Item:

Give your project a name and choose the “Freestyle project” option as the type of the new item.
“Jenkins ‘freestyle’ jobs support simple continuous integration by allowing you to define sequential tasks in an application lifecycle.” — Jenkins documentation
You should now land on the configuration page of your newly created project.
Parameterize Your Project
Select “This project is parameterized” in order to configure the input parameters you want to use for building your application:

The first parameter we want to add is the application name. Click on ADD PARAMETER and select Active Choices Parameter. Let’s name this parameter “app,” which will represent the application we want to build.
For this tutorial, we will hardcode the applications, so we will use the simplest Groovy script you can ever imagine:
return ['first_application', 'second_application']
Once you apply the changes, you should be able to see that the app dropdown is filled with the above applications.
Next, we want to dynamically retrieve the Git branches depending on the selected application. For that, we will add an additional parameter, “branch,” which will consist of all the branches of the selected application.
Click on ADD PARAMETER, and select Active Choices Reactive Parameter:
“The Active Choices plugin is used in parametrized freestyle Jenkins jobs to create scripted, dynamic, and interactive job parameters. Active Choices parameters can be dynamically updated and can be rendered as combo-boxes, check-boxes, radio-buttons or rich HTML UI widgets.” — Jenkins Active Choices documentation
We use this type of parameter since we want to display the branches according to the selected application name, in the previous “app” option. For that, we should tell Jenkins that we want to reference the “app” variable. Add “app” to the “Referenced parameters” option:

Now, let’s write some Groovy! Select the “Groovy script” option and paste the below code on the textbox:
Explanation:
list_branches
is the command we use for listing remote branches of a given repository.awk
andcut
commands are used to extract the specific branch name from the Git command above.- In lines 7–10 we pipe the commands to get the result, split to create an array, and finally the result.
The last parameter we should support is the tag, which will allow us to know which tag we should have on our image. For that, add a new parameter of type String Parameter — a simple free-text input option. Name it “tag.”
Create the Build Script
Now we have all the needed information to compile and build the selected project.
Select “Execute shell” in the Build step:

Pass the following content:
Explanation:
- First clone the selected application.
- Run the build command to compile the source code.
- Package the code using the provided Dockerfile (in the repository itself)
- Publish the image to the hub.
Note: If the two projects are built in the same manner, you can omit the condition and combine them.
Depending on your build tool and source code, the process can be different from the one above. However, what is important to understand here is the process itself, and not specific commands to run.
Conclusion
Using Jenkins, we created a simple job that can be used by anyone who wants to build a specific application from their branch. The process above can be generalized more, and it can be integrated into a bigger pipeline to provide a full CI/CD.