How to Show Snackbars Across Multiple Screens in Jetpack Compose
Provide intuitive feedbacks in your Android apps
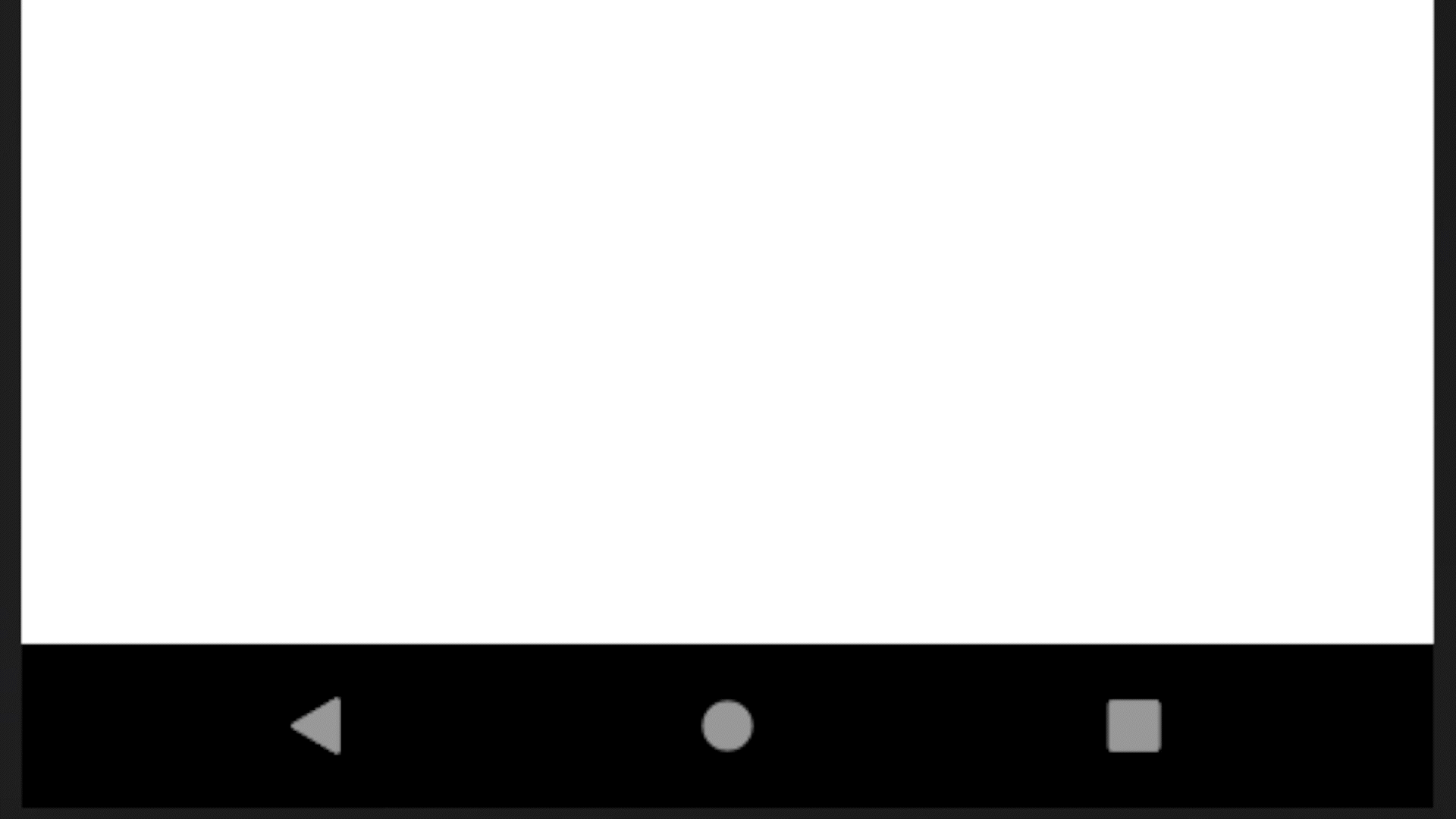
Goal
We want our Snack bars to be visible even when navigating to a new screen in Jetpack Compose. So the Snackbar should be displayed across multiple screens.
TL;DR:
We should keep a scaffoldState in the app state holder, and let the screens trigger a showSnackbar
event.
In this article, I will explain how to achieve showing Snackbars even when navigation is triggered in your Jetpack Compose app. This is small app, but it is very important to use the solutions recommended by Android in order to be able to maintain a bigger project.
The first key point is an app state as a source of truth, explained here: State Holders as Source of Truth.
The second key point is state hoisting, explained here: State Hoisting.
The solution relies on these two things. We will have an app state holder, and our screens will trigger an event notifying our single scaffoldState
that a snackbar should be displayed. Since we only have a single Scaffold (and it’s state, stored in the app state) in our root composable, it will handle not only the display of the Snackbars but it will handle if multiple Snackbars are scheduled, and it will show every one of them that is not canceled.
Let’s walk through the code:
First, we have the MainActivity.kt
— nothing special:
The root composable looks like this:
We can see that we have the state holder injected into our composable, and we use its properties and function(s).
So let’s see how does the SnackbarDemoAppState
looks like.
Here we are collecting everything that our app should remember, then create our own remember. AppState()
composable function. Since it is in our root composable, our Snackbar will not disappear when a screen leaves the composition.
Because we have only one scaffoldState
it will handle every showSnackbar
request, and schedule every message that should be displayed.
What does the MyNavigation
composable do:
It just passes the showSnackbar
on to the screens, so they can call it. (And obviously handles the navigation.)
How does an actual screen look like:
You can see when navigation is triggered, we can also call the showSnackbar
function. We have to provide our message and duration.
Result
Our snack bars are always shown no matter if navigation is called (even the back button clicked).

Summary
We followed the recommended solutions to store state in our app and send down callable events in our composition tree in order to show snack bars even when navigating to new screens in Jetpack Compose.
Full code: https://github.com/Abokyy/snackbardemo-app
Thanks for reading.