How to Make a Simple Game Loop Using Vanilla JavaScript
Build your first game today

Hey everybody! Today I want to talk about how you can create a simple game loop using only vanilla JavaScript. Recently, my friend and I decided to work on a little game project to up our JS skills, and we had to restart a few times until we could get a proper game loop going. So I decided to write a quick tutorial for all you beginner game programmers having a bit of trouble getting started.
Setting Up Your Canvas
In order to follow this tutorial, you are going to need to set up three files. The first is an index.html
file, the second is a script.js
file, and lastly you’ll need a style.css
file.
First things first. If we’re making a game, we are going to inevitably want to draw some stuff on the page. The best way to do this is to use something in HTML called the canvas
tag.
<canvas> id="canvas"></canvas>
The canvas
tag is just a way to draw graphics on the screen using JavaScript and HTML. To use it in our cool new game, we are going to add it to our HTML file like so.
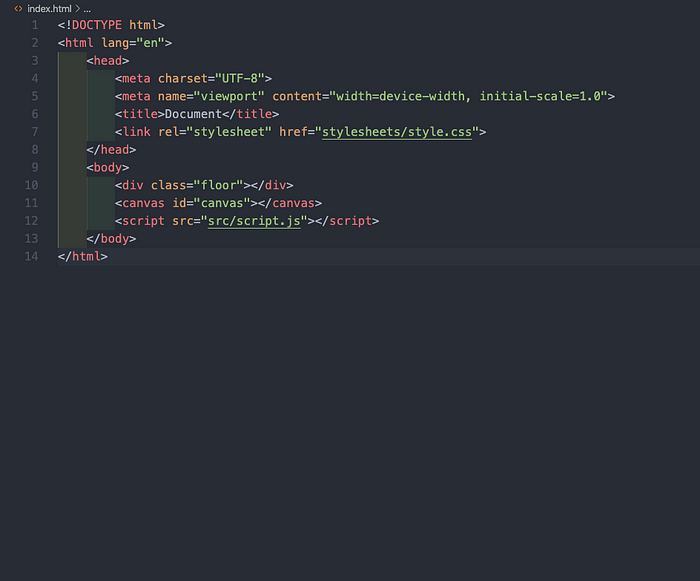
Now that we have our HTML taken care of, we’re going to want to use JavaScript to access our canvas
tag, and alter it. To do so, we will do this:
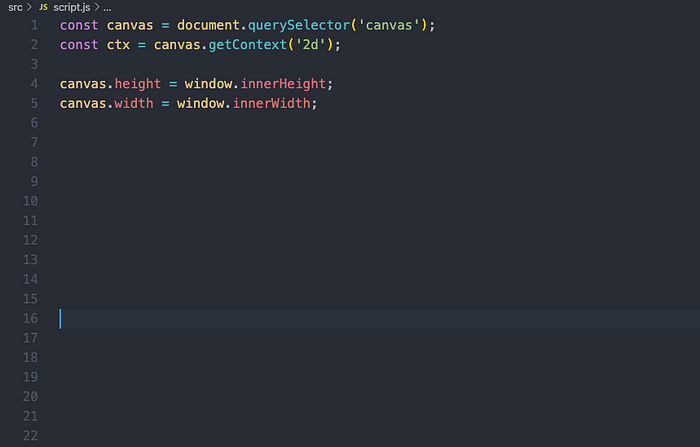
What we are doing here is storing our canvas
tag into a variable called “canvas,” and then we set its context to 2D since we are going to be drawing 2D graphics on our screen. After this, we set the height and width of our canvas to equal our window’s height and width.
Before we move on, let’s jump over to our CSS and quickly style our canvas:

Okay almost done. Hopefully, I haven’t lost you yet. The last thing we are going to do is set up an event listener so that if our user ends up resizing our screen, we can adjust our canvas as well. We’ll do that like this:
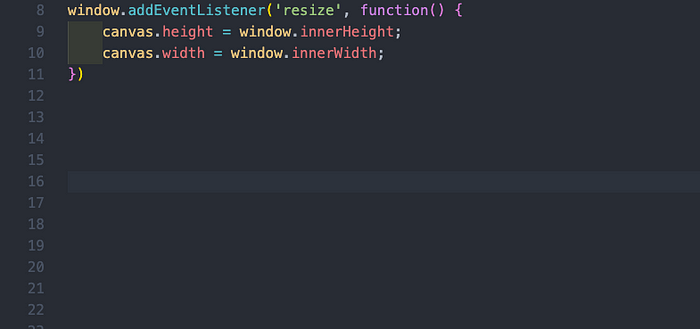
Making the Game Loop
To make a game loop, we need to clear out our canvas and redraw it every frame of our game. This is because in order to get things to move continuously, we have to draw them in new places every frame, and we don’t want the previously drawn sprites or shapes to stick around. So we are going to create an animate
function that will clear out our canvas every frame, like so:

So what are we doing here? Well, first we are making a function called animate
to clear out our canvas . This is essentially our game loop and where you will end up drawing your sprites to the screen. We then use the clearRect
function to completely wipe our canvas. Then when our window loads, we want to start running our game loop.
To do this, we call the setInterval
function, which takes in two parameters. The first is a callback function, which will be the function you want it to execute. And the second is the time interval in which you want it to fire. The interval is in milliseconds, so I put 1000/30 which makes it so our function runs 30 times per second. If you’ve ever played video games before, you’ve probably heard the term 30 frames per second. That’s exactly what we are doing here!
Conclusion
And that’s it. You are now a bonafide game developer. Congratulations! Now you have a simple game loop in which you can create sprites, shapes, platforms and all sorts of cool stuff. Good luck and happy programming.