How To Burn Tokens Using the Anchor Framework on Solana
A brief guide to experimenting with this program
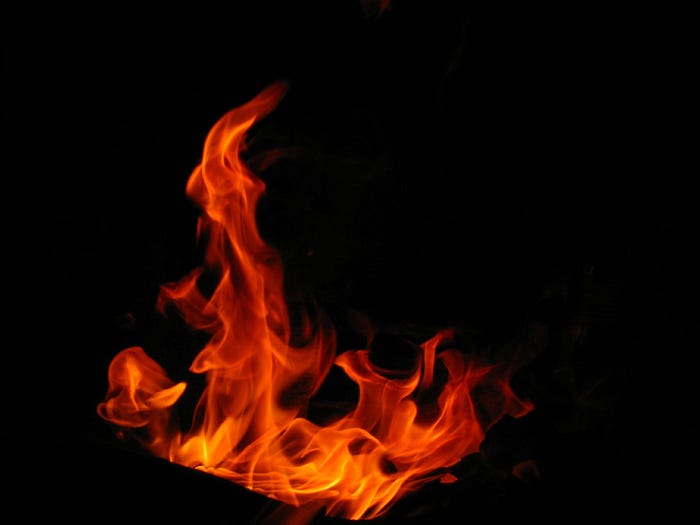
This article is a continuation of the previous article I have written on “How to Initialize Mint Accounts and Mint Tokens Using the Anchor Framework,” found in the following link. I highly recommend reading that article to better understand this tutorial’s background.
This article will show you how to use “derive macros” in the Anchor framework to burn already minted tokens. Before we get started, I want to tell you what the Anchor framework is and how it will help developers like us to build on top of the Solana network. Unlike the Ethereum ecosystem, developing on top of Solana is slightly different, mainly because they are two types of blockchain networks.
If you have previous experience developing on the Solana network, you would know that serialization and deserialization are two concepts you should always keep in mind when developing on Solana. This is where the Anchor framework comes in to save the day. This framework simplifies the serialization/deserialization of structs so that the developers do not need to worry about it. This is done through “derive macros.”
According to the official documentation, as found here, derive macros can provide further functionality through the use of attributes and instructions.
In this article, let’s see how the Anchor framework has made the process of burning tokens from a specific associated token account(ATA) very easy for developers.
Step 1. Prerequisites
- VS Code — Code editor
- Anchor version — 0.24.2
- Part 1 of this tutorial is found here
- Previous knowledge of Anchor framework
Step 2. Approve Tokens To Be Minted by the Owner of ATA
This is the Rust code to transfer approval to another party to burn the tokens owned by someone else.
Let us dive into this code and see how this works:
- The struct
ApproveToken
is what gets passed into the functionapprove_token.
- Line 31 specifies the tokens of which ATA we should approve.
- Line 34 specifies to whom the approval to burn should be given. It is important to note that this transaction should be signed by the current owner of the ATA, where the tokens are now in.
- The
signer
in line 35 should be the owner of the ATA mentioned in line 31.
This struct would then be passed on to the approve_token
function along with the number of tokens the approval should be given to, specified as amount
. Then a cross-program invocation (CPI) would be executed to approve for the tokens to be burned.
Now, let us look at the JS code for granting approval for tokens to be burned.
As you can see in the above piece of code, the functions are signed by fromWalletB
, which currently owns the ATA and the tokens inside it. Since fromWalletA
is specified as the delegate
, after this transaction has been executed, the approval of 100 tokens in the given ATA will be given to fromWalletA
to be burned.
An important thing to notice is that even after the transaction has been executed, the specified amount of tokens will still be inside the same ATA. Only its approval to be burned is transferred to fromWalletA
.
Another important thing to remember is that if you want your tokens to be burned, the approval should be given back to the original owner of the mint account. Even though you transferred the approval to some random wallet, they can’t be burned and removed from your ATA later. Only the owner of the mint account from where the tokens came can burn them.
Step 3. Burn Tokens from an ATA
Assuming the approval of the tokens to be minted has been transferred back to the owner of the mint account, let’s see how we can write the code to burn tokens from a specific ATA.
This code is similar to the code used to mint tokens in the previous tutorial. Therefore, I won’t go deep into this code to explain how it works. It should be pretty obvious to you by now. It is important to note that in lines 34 and 35, the from
account is marked as mut
because the function will change the account's balance. Likewise, the mint
account is also marked mut
. After burning, the total supply of tokens will reduce.
Now, let us see how to interact with this Rust code from JS:
This is also very similar to the JS code for minting tokens. Therefore, I will not waste your time explaining the code. If you have any questions, please feel free to drop a comment.
And there you go. That is how you burn tokens using the Anchor framework. You can see how the Anchor framework has made the life of Solana developers very easy.
I will follow up with some other tutorials from the blockchain world.
Till then,
Cheers!!
Resources
- Anchor Lang document — https://docs.rs/anchor-lang/latest/anchor_lang/derive.Accounts.html
- Part 1 of the tutorial — https://betterprogramming.pub/how-to-initialize-mint-accounts-and-mint-tokens-using-the-anchor-framework-c989d1ba4f97