A Glimpse at Quarkus vs. Gin Gonic
A quick REST API with microservice frameworks

For quite some time now I had the wish to checkout some of the newest and most famous web frameworks. In this article I want to take a look at DevEx for the following technologies.
The Web-Frameworks I want to have a look at:
Requirements
To make this glimpse possible we need a little application that can be built easily but contains the functionality of a modern web app.
That is why I chose — who would have thought — a weather app.
The use can receive weather information for a city he searched for.
The data for it will be coming from a free 3rd Party API using REST.
Same goes for the city search — the API will provide for that.
I am not going to do anything to the data received but simply tunnel the JSON response.
Basic Architecture

Let’s stick to a simple architecture. We have one backend service. This will communicate with the API of openweathermap. The service will then serve the data using a simple REST API to the client.
Our hypothetical Frontend could then simply call GET /weather/:name.
Go with Gin Gonic
I will be using version 1.16.6.
In the documentation you can find a valuable link with a great tutorial for a restful API with Gin. That is exactly what we need!

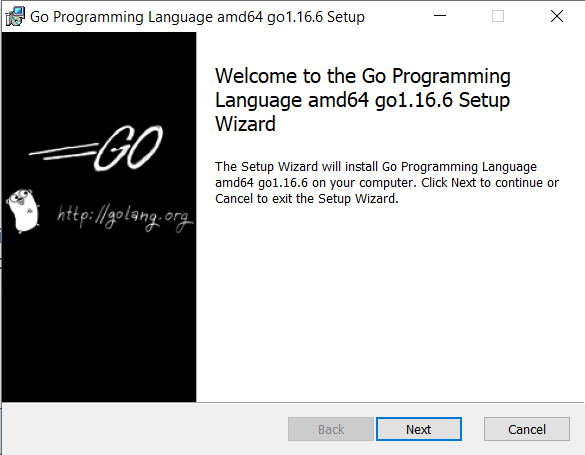
After installation — don’t forget to set the Environment variable with GOPATH.

$ mkdir wetterapp-gin
$ go mod init davidminkovski.com/wetterapp-gin
Let’s create the folder for our weather app and initialize.
I followed the tutorial and created a Test API.
For the IDE I used Visual Studio Code.
package mainimport (
"github.com/gin-gonic/gin"
"net/http"
)type city struct {
ID string `json:"id"`
Name string `json:"name"`
}var cities = []city{
{ID: "1", Name: "Frankfurt am Main"},
{ID: "2", Name: "Köln"},
}func main() {
router := gin.Default()
router.GET("/cities", getCities)
router.Run("localhost:8080")
}func getCities(c *gin.Context) {
c.IndentedJSON(http.StatusOK, cities)
}
Now there are two commands left to execute.
The first one loads dependencies and the second starts the server.
$ go get .
$ go start .

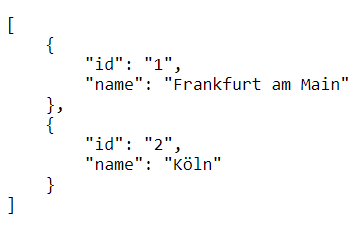
Okay that was super fast. 30 Minutes later and I have my REST API.
Now I want to query the weather API. There you go.
package mainimport (
"github.com/gin-gonic/gin"
"io/ioutil"
"log"
"net/http"
)func main() {
router := gin.Default()
router.GET("/weather/:name", getWeatherForCity)
router.Run("localhost:8080")
}func getWeatherForCity(c *gin.Context) {
name := c.Param("name")
key := "MY_API_KEY"res, err := http.Get("http://api.openweathermap.org/data/2.5/weather?q=" + name + "&appid=" + key)
if err != nil {
log.Fatal(err)
}
responseData, err := ioutil.ReadAll(res.Body)
if err != nil {
log.Fatal(err)
}
c.Data(http.StatusOK, "application/json", responseData)}

That was it! I have a simple Web API Server that I can use to query weather data for a city name. I must say coming from a JS or Java background, it took a some time to understand and get used to the new syntax and language features, but wow this SPEED!
Rating
- Ease of Use: 1/3
- Speed: 3/3
- Documentation: 2/3
Java with Quarkus
I will use Quarkus version 2.1.0.


First of all Quarkus runs on the GraalVM and you need the JDK for Java.
Then we will need the package managers maven or gradle. This takes around 15 minutes — again, don’t forget to setup the right environment variables.
Quarkus really shines with its Configurator. Similar to other Java Frameworks like SpringBoot, you can pick the right packages and simply generate the needed application. Unfortunately it is not super intuitive to know which one is which and for what. I went with my gut and chose the following:


After a quick download I have my project.
It comes with a basic setup, readme and docker configurations.

The documentation provides a great article for a REST API. Additionally you will find here enough informationen for working with other APIs.
First of all let us create a model for our weather data.
// WeatherResponse.java@JsonIgnoreProperties(ignoreUnknown = true)
public class WeatherResponse {
public String name;
public Weather alpha2Code;
public Main main;
public Wind wind;
public Coord coord;
public static class Weather {
public String main;
public String description;
public String icon;
}
public static class Wind {
public float speed;
}
public static class Main{
public float temp;
public float feels_like;
public float temp_min;
public float temp_max;
public int pressure;
public int humidity;
}
public static class Coord{
public float lon;
public float lat;
}
}
Now we need a service that will communicate with the 3rd party API.
In the application.properties we set the URL and remove SSL.
// WeatherService.java@Path("/data/2.5/")
@RegisterRestClient(configKey="weather-api")
public interface WeatherService {
@GET
@Path("/weather")
Set<WeatherResponse> getByCity(@QueryParam("q") String name, @QueryParam("appid") String appId);
}// application.propertiesweather-api/mp-rest/url=http://api.openweathermap.org/ #
weather-api/mp-rest/scope=javax.inject.Singleton #
quarkus.tls.trust-all=true
Let’s go ahead and create a resource that will listen to any incoming request.
// WeatherResource.java@Path("/weather")
public class WeatherResource {
@Inject
@RestClient
WeatherService weatherService;
String appId = "MY_API_KEY";
@GET
@Path("/{name}")
@Produces("application/json")
public Set<WeatherResponse> name(@PathParam("name") String name) {
return weatherService.getByCity(name, appId);
}
}
We are done! Coming from a Java background the ease of use here is clearly a factor, but the amount of work one has to do is a lot compared to other frameworks and languages.
Rating
- Ease of Use: 2/3
- Speed: 1/3
- Documentation: 2/3
Summary
I hope this article gave you a little glimpse at Gin Gonic and Quarkus.
Both frameworks are great and have a great future ahead.
Especially being Golang and Java frameworks I am sure they will continue to evolve as the programming languages do.
Looking at ongoing microservice trends, these frameworks could be a great base to start your next microservice project!
Curious about more?
My newsletter is a burst of tech inspiration, problem-solving hacks, and entrepreneurial spirit.
Subscribe for your weekly dose of innovation and mind-freeing insights:
https://davidthetechie.substack.com/