Member-only story
How to Unit Test a GORM Application With Sqlmock
Tips and tricks to work with GROM and PostgreSQL
update at 2022.07: My opinion has changed a bit in the last 2 years, check my latest article on this topic as well if you are interested.
Unit testing DB-interactive codes is not easy. It becomes harder when an ORM library like GORM is involved.
Theoretically, we could mock all the interfaces of database/sql/driver
(such as Conn and Driver) with the great mock tool GoMock. However, we still need a lot of manual work to complete this kind of testing even with the help of GoMock.
The good news is Sqlmock could solve the aforementioned problem. As declared by its official website, it’s an “Sql mock driver for golang to test database interactions.”
This article will show you how to unit test a simple blog application with Sqlmock. The application is backed with PostgreSQL and uses GORM to simplify O-R mapping.
We’ll write test cases with the BDD testing framework Ginkgo, but you can change to any other testing library you prefer.
Our blog application will contain a blog data model and a repository struct to handle DB operations.
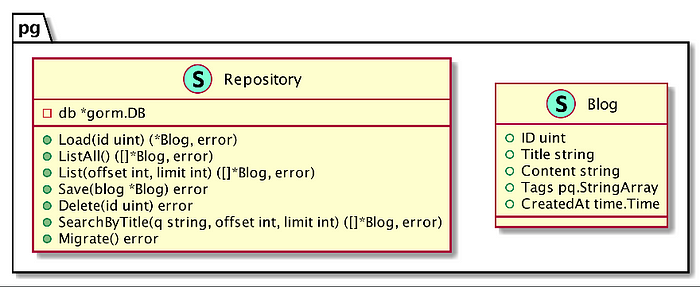
Define GORM Data Model and Repository
Let’s define the blog data model and the repository struct first:
Note the type of Blog.Tags
is pq.StringArray
, which represents string array in PostgreSQL.
Our Repository
struct is pretty simple. It has a field of *gorm.DB
, and all DB operations depend on this field. I have omitted some code for brevity. There are several other methods are declared in the Repository
struct besides Load
and ListAll
, such as Save
, Delete
, SearchByTitle
, etc. These methods will be explained later in this article.